Code Snippet: Oxygen Page Width in Gutenberg Editor
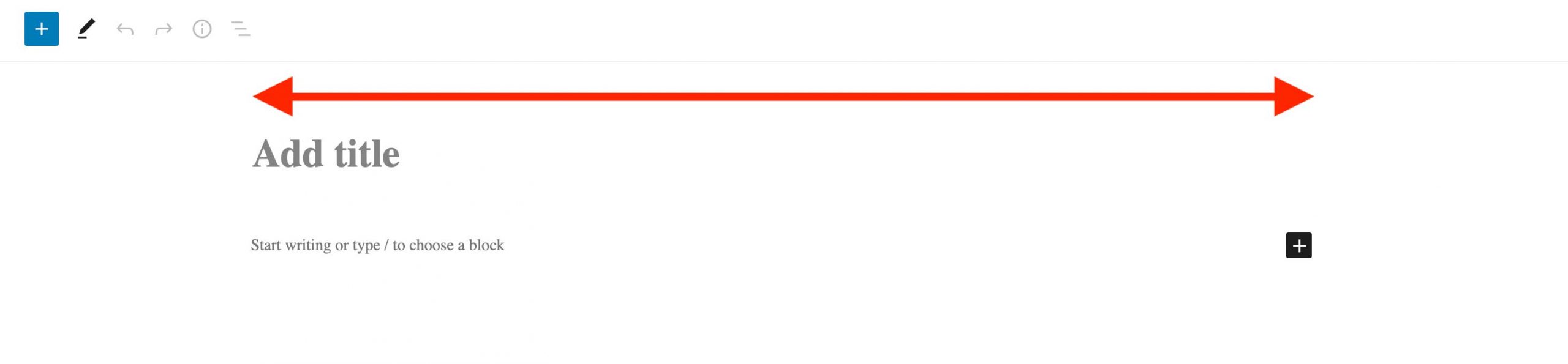
Task
Oxygen Builder allows to define the page width both in the global settings and individually in single templates.
Gutenberg Editor has a default width of 580 pixels.
This is fine for editing simple text posts.
However, if more complex content is to be designed, such as columns or blocks arranged side by side, the narrow Gutenberg Editor is a hindrance.
Solution (Approach)
The code snippet presented here determines the page width defined in Oxygen and displays the Gutenberg Editor in this width.
The snippet first determines the page width defined in the global Oxygen settings.
Then it checks if a different page width is defined for the currently used template. The snippet takes into account the standard templates (start page, blog archive, page and post templates, search results, …) as well as templates individually assigned to the current page.
The determined page width is then applied to the Gutenberg Editor via CSS adjustment.
Please note that the snippet only determines the defined page width. Sidebars, margins or paddings defined in the template cannot be determined.
The page width defined for the Gutenberg Editor does not correspond to a pixel-exact representation. However, the changed page width allows a better assessment of the available space during page editing.
Download
The Code Snippet is available for download here:
oxygen-adapt-gutenberg-editor-width.code-snippets.json
Version 1.0.1, 2020-12-10
For installation and use of the downloaded JSON file you will need the plugin Code Snippets.
You can install the JSON file using the "Import" function of the plugin.
Don't forget to activate the snippet after import.
Alternative: At the end of this page you can view and copy the complete source code of the snippet.
New functionalities and bug fixes are documented in the change log.
Donation
I enjoy developing code snippets and solving requirements with them. I provide the snippets free of charge.
If you like, you can honor my many hours of work with a small coffee donation via PayPal.
Your donation will of course be taxed properly by me.
Performance
While developing the script I paid a lot of attention to efficiency and performance.
The snippet is only called when creating new or editing existing posts or pages.
Both the global Oxygen settings and the post or page data were already read from the database at that time.
The only additional data that needs to be retrieved from the database is the meta data for the actual applicable template.
This should not have a noticeable effect on speed.
Disclaimer
I have developed and tested the code snippet to the best of my knowledge under WordPress 5.5.3 and Oxygen 3.6.
I provide the code snippet for free use.
I cannot give a guarantee for the functionality in all conceivable WordPress environments.
Download and use of this code snippet is at your own risk and responsibility.
Change Log
Version 1.0.0 (2020-11-16)
- Initial Release for the Oxygen community
Version 1.0.1 (2020-12-10)
- Bug Bixes:
- Removed alert script (left behind by mistake!) and optimized editor ready detection
(Thanks to Nico Weinreich for reporting and the improved script!)
- Removed alert script (left behind by mistake!) and optimized editor ready detection
Source Code
<?php /* Project: Code Snippet: Apply Oxygen page width to Gutenberg editor Version: 1.0.1 Description: en: https://www.altmann.de/en/blog-en/code-snippet-oxygen-page-width-in-gutenberg-editor/ de: https://www.altmann.de/blog/code-snippet-oxygen-seitenbreite-im-gutenberg-editor/ Author: Matthias Altmann (https://www.altmann.de/) Copyright: © 2020, Matthias Altmann ============================================================================================================== Description ============================================================================================================== Gutenberg editor has a default page width of 580 pixels. While that is fine for standard blogging websites, it's difficult to create page content without having a clue of the actual available page width. This Code Snippet tries to evaluate the actual page width from Oxygen settings and changes the Gutenberg editor width accordingly. Page Width Evaluation: 1) Read global page width from global Oxygen settings 2) Detect specific template for this page - either any type of special template like front page, archive, etc. - or the template assigned manually to this page, and check for template specific page width 3) Emit some CSS to adapt Gutenberg editor to the max-width evaluated by the previous steps. Limitations: This snippet reads the page width defined in global settings or from a specific template and applies that page width to the Gutenberg editor. The snippet DOES NOT detect any paddings, margins, sidebars etc. in the actual template that might influence the final available space. That means the width defined for the Gutenberg editor is only a better guessing of available space and sure not pixel perfect. Do not rely on the width of the Gutenberg editor for pixel perfect design but use it as a rough frame to get a better idea of the available width while editing. Performance: The snippet is only called when creating a new or editing an existing page or post. The Oxygen global settings as well as the post details have already been retrieved from the database at this point. The only details we still have to read from the database is the metadata for the page specific template. There should be no noticeable performance impact. Version History: Date Version Description -------------------------------------------------------------------------------------------------------------- 2020-11-16 1.0.0 Initial Release for the Oxygen community 2020-12-10 1.0.1 Bug Bixes: - Removed alert script (left behind by mistake!) and optimized editor ready detection (Thanks to Nico Weinreich for reporting and the improved script!) */ add_action('admin_head-post.php', 'maltmann_oxygen_gutenberg_editor_width'); add_action('admin_head-post-new.php', 'maltmann_oxygen_gutenberg_editor_width'); function maltmann_oxygen_gutenberg_editor_width() { global $post; // exit if we don't have a post object if (!isset($post)) return; $width = null; // check Oxygen global settings for a defined page width $ct_global_settings = maybe_unserialize( get_option('ct_global_settings') ); if ($ct_global_settings && is_array($ct_global_settings) && array_key_exists('max-width',$ct_global_settings)) { $width = $ct_global_settings['max-width'].'px'; } $post_id = null; $template = null; // get archive template if ( is_archive() || is_search() || is_404() || is_home() || is_front_page() ) { if ( is_front_page() ) { $post_id = get_option('page_on_front'); } else if ( is_home() ) { $post_id = get_option('page_for_posts'); } else { $template = ct_get_archives_template(); } } if ($post_id || (!$template)) { if (!$post_id) $post_id = $post->ID; // check post specific settings $ct_other_template = get_post_meta($post_id, 'ct_other_template', true ); if (!empty($ct_other_template) && $ct_other_template > 0) { // specific template // try getting specific template $template = get_post($ct_other_template); } elseif ($ct_other_template != -1) { // try getting default template if not explicitly set to not use any template at all if ( intval($post_id) == intval(get_option('page_on_front')) || intval($post_id) == intval(get_option('page_for_posts'))) { $template = ct_get_archives_template( $post_id ); if(!$template) { // if not template is set to apply to front page or blog posts page, then use the generic page template, as these are pages $template = ct_get_posts_template( $post_id ); } } else { $template = ct_get_posts_template( $post_id ); } } elseif ($ct_other_template == -1) { // -1 = no template! $width = 'none'; } } else { // get default archives template $template = ct_get_archives_template(); } if ($template) { $template_meta = maybe_unserialize( get_post_meta($template->ID, 'ct_page_settings', true ) ); if ($template_meta && is_array($template_meta) && array_key_exists('max-width',$template_meta)) { if (!empty($template_meta['max-width'])) $width = $template_meta['max-width'].'px'; } } if ($width) { // style inspired by plugin "Editor Full Width Gutenberg" (https://wordpress.org/plugins/editor-full-width/) echo sprintf('<style id="maltmann-gutenberg-editor-width-style"> body.gutenberg-editor-page .editor-post-title__block, body.gutenberg-editor-page .editor-default-block-appender, body.gutenberg-editor-page .editor-block-list__block { max-width: %1$s !important; } .block-editor__container .wp-block { max-width: %1$s !important; } /*code editor*/ .edit-post-text-editor__body { max-width: %1$s !important; margin-left: 2%%; margin-right: 2%%; } </style>',$width); $script = <<<MALTMANN_END_OF_SCRIPT <script id="maltmann-gutenberg-editor-width-script"> let blockLoaded = false; let blockLoadedInterval = setInterval(function () { // post-title-0 is ID of Post Title textarea if (document.getElementById('post-title-0')) { var widthHint = jQuery('<div style="display:inline-block;">Editor width adapted to %s.</div>'); jQuery('.edit-post-header-toolbar').append(widthHint); blockLoaded = true; } if (blockLoaded) { clearInterval(blockLoadedInterval); } }, 500); </script> MALTMANN_END_OF_SCRIPT; echo sprintf($script,$width); } }